An intuitive roadmap to Python basics: Your first steps in the world of data science.
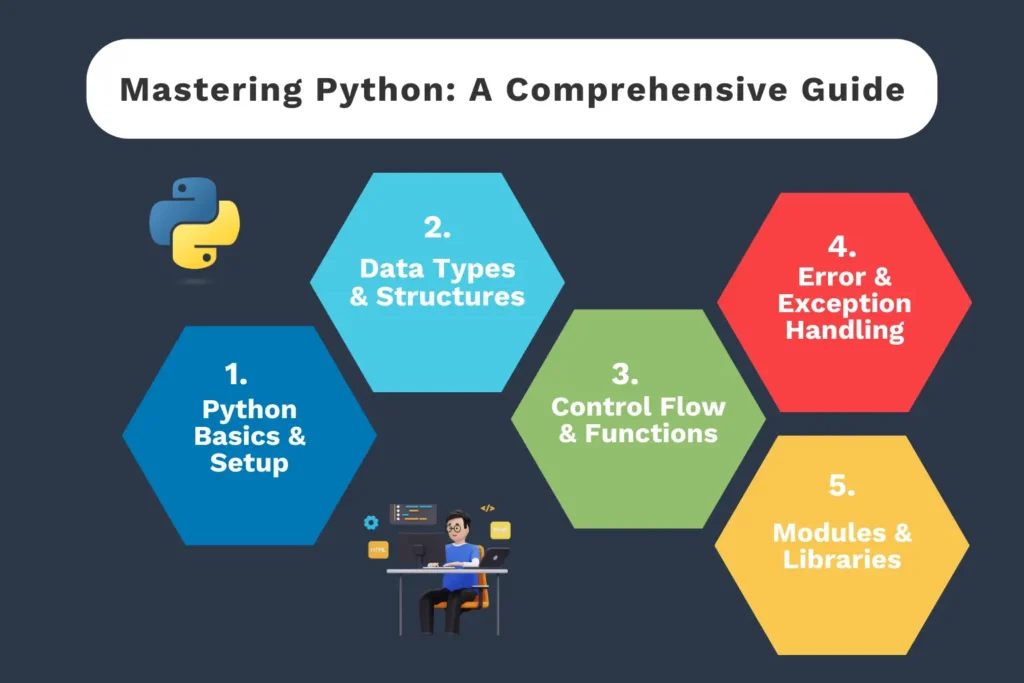
1 . Introduction
Python is an extremely user-friendly language known for its simplicity and readability. This high-level, interpreted programming language plays a vital role in the field of data science due to its flexibility and wide range of applications. Through this article, we aim to teach you the basics of Python that form the stepping stones for becoming proficient in data science.
This tutorial sets out to introduce you to Python at a fundamental level, covering everything from basic syntax and data types to more complex concepts such as control flow, functions, and error handling. We’ve aimed to explain these concepts in a clear, straightforward manner, using plenty of examples to illustrate how they work in practice.
This guide isn’t just for beginners, though. Even if you’re a seasoned professional in another language, you’ll find that Python’s focus on readability and simplicity can make your coding life easier, and this tutorial can serve as a valuable starting point or a refresher.
So, let’s embark on this exciting journey of learning Python together! Welcome aboard, and happy coding!
Before starting the article, here are the topics we covered.
Table of Contents
- Introduction
- Understanding Python Basics
- Python Data Types
- Built-in Functions in Python
- Control Flow: If Statements and Loops
- Functions in Python
- Handling Errors and Exceptions in Python
- Python Modules and Libraries
- Conclusion
- References
1.1 What is Python?
Python, a high-level interpreted programming language, was created by Guido van Rossum and first released in 1991. It was designed with readability in mind, which is evident in its use of significant white-space.
Over time, Python has made an indelible mark in various fields, including data science, artificial intelligence, and web development. In layman’s terms, it means that Python is a language that is easy to understand and write (high-level) and can be run directly line by line (interpreted).
1.2 Installing Python and Setting Up Your Environment
For this tutorial, we’ll be using Google Colab, an online platform that allows you to write and execute Python right in your browser. It is an excellent tool, especially for those just starting their Python journey, as it requires no setup, offers free access to computing resources, and even provides many useful features for writing and sharing your code.
To get started with Google Colab, simply click this link. If you’re new to the platform, I recommend checking out this Introduction to Colab to get acquainted with its features.
Of course, if you prefer a local development environment, feel free to set up Python and Jupyter Notebook on your machine. Anaconda is a great distribution for this, as it includes Python, Jupyter, and a host of other tools.
For a more interactive learning experience, I’ll be providing a Python Notebook on my GitHub repository. This will enable you to directly use the notebook on Google Colab or Anaconda, making it easier to follow the tutorial. Be sure to check the final section of this article for the GitHub link.
Remember, the environment isn’t as important as the code you’re writing and the concepts you’re learning. Choose the tool that makes you feel most comfortable and lets you focus on learning Python. Now, let’s dive in!
2 . Understanding Python Basics
2.1 Printing Output:
The print()
function is used to output text, numbers, or the result of operations to the console. For example:
print("Hello World!")
Output:
Hello World!
In this example, the print()
functions prints the specified message to the screen or other standard output device. So it will display Hello World!
In Python, you can define a string using either single (‘ ‘) or double (“ “) quotes. For instance:
print('Hello, Python!')
print("Hello, Python!")
Output:
Hello, Python!
Hello, Python!
Both of these statements will output ‘Hello, Python!’. Python treats single quotes the same as double quotes. This flexibility allows us to easily include quotes as part of our string. For example:
print("It's a beautiful day!")
Output:
It's a beautiful day!
In the above statement, we used double quotes for the string because the text contains an apostrophe, which is a single quote.”
2.2 Comments:
A very crucial part of writing good, understandable code is the use of comments. Comments in Python start with the hash character (#
) and extend to the end of the physical line. They are used to explain what a piece of code is supposed to do, making it easier for others (and your future self) to understand your code.
Python ignores comments, and they have no impact on the execution of the program. This means you can write comments without worrying about how they might change your code.
Here’s an example of a single-line comment:
# This is a comment and Python will ignore this line.
print("Hello, World!")
Output:
Hello, World!
In this example, Python will only execute the print
statement. The text after the ‘#
‘ on the first line is a comment and is ignored by Python.
For longer comments that span multiple lines, you can use a ‘#
‘ on each line:
# This is a multi-line comment.
# Each line starts with a '#'.
# Python will ignore these lines.
print("Hello, World!")
Output:
Hello, World!
Python also allows for multi-line strings which are not assigned to a variable to serve as a multi-line comment, although this is less conventional:
'''
This is another way to write
multi-line comments in Python.
Python will ignore these lines.
'''
print("Hello, World!")
Output:
Hello, World!
2.3. Variables:
Variables are like containers where you store values or data. They can be assigned any value using the equal ‘=
‘ sign. For example:
# Storing a number
age = 20
print(age) # Output: 20
# Storing a string
name = "John"
print(name) # Output: John
# Storing the result of an operation
sum = 10 + 15
print(sum) # Output: 25
Output:
20
John
25
2.4 Naming Conventions for Variables
In Python, while you can name your variables almost anything, there are few rules and best practices to follow.
Rule 1: Variable names should start with a letter or an underscore, but not with a number.
* Correct: my_var, _var
* Incorrect`: 9var
Rule 2: Variable names can only contain alphanumeric characters and underscores (A-z, 0–9, and _ )
* Correct: my_var1
* Incorrect: my-var, my$var, my var
Rule 3: Variable names are case-sensitive (var, Var, and VAR are all different variables)
Best Practices:
- Use meaningful names that indicate the purpose of the variable. Instead of x, use age, or name.
- For multi-word variable names, use underscores to separate the words for readability. For example, user_input, speed_limit.
# Correct Variable Naming
user_name = "Alex"
user_age = 30
_total = 100
# Incorrect Variable Naming
9name = "Alex" # starts with a number
total$ = 100 # contains special character
Output:
Input In [6]
9name = "Alex" # starts with a number
^
SyntaxError: invalid syntax
2.5 String Formatting — f-string
When working with strings in Python, you often need to include variable values within the string itself. For this, Python provides an extremely convenient method known as f-string formatting.
An f-string is a string literal that is prefixed with ‘f
‘. The expressions within the curly braces {}
are replaced with their values. This allows you to embed expressions inside string literals, using curly braces {}
.
Let’s look at an example:
name = "Alice"
age = 15
# using f-string to print value within the string
print(f"Hello, my name is {name} and I am {age} years old.")
Output:
Hello, my name is Alice and I am 15 years old.
When you run this code, Python replaces {name}
with the value of the variable name, and {age}
with the value of age.
2.6 Comparison and Assignment Operators
Comparison and assignment operators are essential in Python as they allow us to compare and assign values.
=
is an assignment operator. It’s used to assign the value on the right to the variable on the left. For example:
x = 5
Here, 5 is assigned to the variable x
.
==
is a comparison operator that checks whether the values of two operands are equal. If the values are equal, the condition evaluates toTrue
; otherwise, it evaluates toFalse
. For example:”
x = 5
print(x == 5)
Output:
True
This will output True
because x
is indeed 5.
!=
is also a comparison operator. It checks if the values of two operands are not equal. If they are not equal, the condition becomes true. For example:
x = 5
print(x != 5)
Output:
False
This will output False
because x
is not equal to 5 is False
statement.
These are just a few examples of comparison and assignment operators in Python. Understanding these will allow you to write more complex and interesting programs.
3. Python Data Types
3.1. Numbers
In Python, numbers can be of three types: integers (like 3, 5, 7), floating-point numbers (like 3.14, 6.022), and complex numbers (like 1 + 2j). Here’s an explanation of the three primary numeric data types in Python, along with their main operations:
3.1.1. Integers:
These are positive or negative whole numbers with no decimal point. Integer size is limited only by your machine’s memory.
Example:
x = 10
y = -5
print(type(x)) # Output: <class 'int'>
Output:
<class 'int'>
3.1.2. Floats:
Floating point numbers in Python are notable for their decimal point. They can also be scientific numbers with an “e” or “E” to indicate the power of 10.
Example:
x = 20.5
y = -13.4
z = 35e3 # 35000.0, the 'e' denotes 'times 10 raised to'
print(type(x)) # Output: <class 'float'>
Output:
<class 'float'>
3.1.3. Complex Numbers:
Complex numbers are written with a “j” or “J” as the imaginary part.
Example:
x = 3+5j
print(type(x)) # Output: <class 'complex'>
Output:
<class 'complex'>
Main operations
Addition (+): Adds values on either side of the operator.
x = 5
y = 3.5
print(x + y) # Output: 8.5
Output:
8.5
Subtraction (-): Subtracts right-hand operand from left-hand operand.
x = 10
y = 6
print(x - y) # Output: 4
Output:
4
Multiplication (*): Multiplies values on either side of the operator.
x = 7
y = 2
print(x * y) # Output: 14
Output:
14
Division (/): Divides left-hand operand by right-hand operand.
x = 20
y = 5
print(x / y) # Output: 4.0
Output:
4.0
Modulus (%): Divides left-hand operand by right-hand operand and returns the remainder.
x = 10
y = 3
print(x % y) # Output: 1
Output:
1
Exponentiation (**): Performs exponential (power) calculation on operators.
x = 2
y = 3
print(x ** y) # Output: 8
Output:
8
Floor Division (//): The division of operands where the result is the quotient in which the digits after the decimal point are removed.
x = 17
y = 4
print(x // y) # Output: 4
Output:
4
3.2 Strings
Strings in Python are sequences of characters and are defined either with a single quote ‘ ‘ or a double quote “ “. Strings in Python are immutable, which means once defined, you cannot change their contents.
str1 = 'Hello,'
str2 = " World!"
print(type(str1)) # Output: <class 'str'>
Output:
<class 'str'>
3.2.1 String Operations:
Concatenation: Concatenation means to link two things together. In Python, you can concatenate strings using the + operator.
str1 = 'Hello,'
str2 = " World!"
str3 = str1 + str2 # Concatenating str1 and str2
print(str3) # Output: Hello, World!
Output:
Hello, World!
Slicing: Slicing is an operation to extract a part of the string. In Python, we can slice strings using the slice operator [] and [:].
str1 = "Hello, World!"
print(str1[7:12]) # Slice from index 7 to 11, Output: World
print(str1[7:]) # Slice from index 7 to the end, Output: World!
print(str1[:5]) # Slice from the start to index 4, Output: Hello
print(str1[:-1]) # Slice from the start to the second last character, Output: Hello, World
Output:
World
World!
Hello
Hello, World
Indexing: Indexing is used to access individual characters in the string. Python uses zero-based indexing, i.e., indexing starts from 0.
str1 = "Hello, World!"
print(str1[0]) # Output: H
print(str1[7]) # Output: W
Output:
H
W
Also note, Python supports negative indexing, where -1 refers to the last character, -2 refers to the second last character and so on.
str1 = "Hello, World!"
print(str1[-1]) # Output: !
print(str1[-2]) # Output: d
Output:
!
d
3.2.2 Escape Sequences in Strings
To include special characters in strings, Python uses the backslash (\
) as an escape character. For instance, if you want to include a quote within a string that is surrounded by the same type of quotes, you can use the \"
(or \'
for single quotes) escape sequence:
print("She said, \"Hello, World!\"")
Output:
She said, "Hello, World!"
Similarly, to include a new line in a string, you can use the \n
escape sequence:
print("Hello,\nWorld!")
Output:
Hello,
World!
In addition to the new line (\n
), Python offers another handy escape sequence, the tab (\t
). This sequence creates a space equivalent to a tab in your text:
print("Hello,\tWorld!")
Output:
Hello, World!
As you can see, a tab space was added between ‘Hello,’ and ‘World!’.
Escape sequences such as these can be incredibly useful for formatting output in Python. Now, with the understanding of string manipulation and escape sequences, you are equipped to create more complex and dynamic messages in your Python programs!”
3.3 Boolean Data Type
The Boolean data type in Python consists of two values — True
or False
. They often emerge when comparing variables or values.
print(10 > 5) # Output: True
Output:
True
In this case, Python evaluates if 10 is greater than 5, and since it’s true, Python returns True
.
You can also directly assign Boolean values to variables:
is_python_fun = True
print(is_python_fun) # Output: True
Output:
True
Here, the variable is_python_fun
is assigned the Boolean value True
. You’ll use Boolean extensively in control flow and logical operations in Python.
3.4 Lists:
A list in Python is an ordered collection of items that can be of any type. Lists are similar to arrays in other languages, but with more powerful features. Lists are mutable, meaning that you can change their content without changing their identity. You can recognize lists by their square brackets []
that hold elements, separated by a comma ,
.
my_list = [1, "Hello", 3.14, [1, 2, 3]]
print(type(my_list)) # Output: <class 'list'>
Output:
<class 'list'>
In the example above, my_list
is a list containing an integer, a string, a floating-point number, and another list.
3.4.1 Operations with Lists:
Creation: You can create a list by placing a comma-separated sequence of items inside square brackets []
.
my_list = ['apple', 'banana', 'cherry']
Adding Elements: Elements can be added to a list with the append()
method, or you can concatenate two lists with the +
operator.
my_list = ['apple', 'banana', 'cherry']
my_list.append('date') # Using append() to add an element
print(my_list) # Output: ['apple', 'banana', 'cherry', 'date']
my_other_list = ['elderberry', 'fig']
my_list = my_list + my_other_list # Concatenating two lists
print(my_list) # Output: ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
Output:
['apple', 'banana', 'cherry', 'date']
['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
Removing Elements: Elements can be removed from the list using the remove()
method, or the del
statement if you know the index of the element.
my_list = ['apple', 'banana', 'cherry']
my_list.remove('banana') # Remove an item by value
print(my_list) # Output: ['apple', 'cherry']
del my_list[0] # Remove an item by index
print(my_list) # Output: ['cherry']
Output:
['apple', 'cherry']
['cherry']
Accessing Elements: List elements can be accessed by their index, using the slice operator []
. Python uses zero-based indexing.
my_list = ['apple', 'banana', 'cherry']
print(my_list[0]) # Output: apple
# Accessing list elements with negative indexing
print(my_list[-1]) # Output: cherry
Output:
apple
cherry
Also, similar to strings, we can use slicing to access a range of list elements.
my_list = ['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
print(my_list[2:5]) # Output: ['cherry', 'date', 'elderberry']
Output:
['apple', 'banana', 'cherry', 'date', 'elderberry', 'fig']
['cherry', 'date', 'elderberry']
3.5 Tuples
A tuple in Python is similar to a list. It is a collection of elements, which can be of different types. The elements of a tuple are ordered and unchangeable. Tuples are written with round brackets ()
.
3.5.1 Comparison with Lists
Unlike lists, once a tuple is created, you cannot change its contents — no appending, removing, or modifying elements. This might seem like a disadvantage, but it’s not necessarily so. When you’re dealing with a sequence of elements that you know won’t change, using a tuple instead of a list can be more efficient in terms of memory usage and performance.
my_tuple = (1, "Hello", 3.14, [1, 2, 3])
print(type(my_tuple)) # Output: <class 'tuple'>
Output:
<class 'tuple'>
In the example above, my_tuple
is a tuple containing an integer, a string, a floating-point number, and a list.
3.5.2 Operations with Tuples:
Creation: You can create a tuple by placing a comma-separated sequence of items inside round brackets ()
.
my_tuple = ('apple', 'banana', 'cherry')
Adding Elements: Technically, because tuples are immutable, you cannot add elements to a tuple. However, there’s a workaround by concatenating two tuples using the +
operator
my_tuple = ('apple', 'banana', 'cherry')
my_other_tuple = ('date', 'elderberry')
my_tuple = my_tuple + my_other_tuple
print(my_tuple) # Output: ('apple', 'banana', 'cherry', 'date', 'elderberry')
Output:
('apple', 'banana', 'cherry', 'date', 'elderberry')
Removing Elements: Similar to adding elements, you cannot remove elements from a tuple because tuples are immutable. Instead, you could convert the tuple to a list, remove the element, and convert it back to a tuple.
my_tuple = ('apple', 'banana', 'cherry')
my_list = list(my_tuple) # Converting the tuple to a list
my_list.remove('banana') # Remove an item
my_tuple = tuple(my_list) # Convert the list back into a tuple
print(my_tuple) # Output: ('apple', 'cherry')
Output:
('apple', 'cherry')
Accessing Elements: Similar to lists, you can access tuple elements by their index, using the slice operator []
. Python uses zero-based indexing.
my_tuple = ('apple', 'banana', 'cherry')
print(my_tuple[0]) # Output: apple
# Accessing tuple elements with negative indexing
print(my_tuple[-1]) # Output: cherry
Output:
apple
cherry
Also, similar to lists, you can use slicing to access a range of tuple elements.
my_tuple = ('apple', 'banana', 'cherry', 'date', 'elderberry', 'fig')
print(my_tuple[2:5]) # Output: ('cherry', 'date', 'elderberry')
Output:
('cherry', 'date', 'elderberry')
3.6 Set:
A set in Python is an unordered collection of unique items. We define a set with curly braces {}
and separate items with commas. If you want to create an empty set, use set()
.
# A set of integers
my_set = {1, 2, 3}
print(my_set) # Output: {1, 2, 3}
# An empty set
empty_set = set()
print(empty_set) # Output: set()
Output:
{1, 2, 3}
set()
One of the key features of a set is that it doesn’t allow duplicates. If we try to add a duplicate item to a set, Python ignores it.
# A set with duplicate items
my_set = {1, 2, 2, 3, 3, 3}
print(my_set) # Output: {1, 2, 3}
Output:
{1, 2, 3}
3.6.1 Set Operations
Union Operation: The union operation is used to merge two sets together. The resulting set will include all unique elements from both sets.
# Initialize two sets
set_a = {1, 2, 3, 4, 5}
set_b = {4, 5, 6, 7, 8}
# Perform a union operation
set_union = set_a.union(set_b)
print(set_union) # Output: {1, 2, 3, 4, 5, 6, 7, 8}
Output:
{1, 2, 3, 4, 5, 6, 7, 8}
Intersection Operation: The intersection operation will return a new set containing only the common elements of both sets.
# Perform an intersection operation
set_intersection = set_a.intersection(set_b)
print(set_intersection) # Output: {4, 5}
Output:
{4, 5}
Difference Operation: The difference operation will subtract one set from another and return the unique elements of the first set.
# Perform a difference operation
set_difference = set_a.difference(set_b)
print(set_difference) # Output: {1, 2, 3}
Output:
{1, 2, 3}
Symmetric Difference Operation: The symmetric difference operation returns all elements that are unique to each set.
# Perform a symmetric difference operation
set_sym_difference = set_a.symmetric_difference(set_b)
print(set_sym_difference) # Output: {1, 2, 3, 6, 7, 8}
Output:
{1, 2, 3, 6, 7, 8}
In each example, you can see how these operations interact with the sets set_a
and set_b
. Note that these operations do not modify the original sets; they create and return new ones.
3.7 Dictionaries:
A dictionary in Python is an unordered collection of items. Each item stored in a dictionary has a key-value pair. You can use the key to access the corresponding value. Dictionaries are mutable, meaning that you can add or remove items. Dictionaries are written with curly brackets {}
.
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
print(type(my_dict)) # Output: <class 'dict'>
Output:
<class 'dict'>
In the example above, my_dict
is a dictionary with keys name
, age
, city
and corresponding values Alice
, 25
, New York
.
3.7.1 Operations with Dictionaries:
Creation: You can create a dictionary by placing a comma-separated sequence of key-value pairs inside curly brackets {}. Key-value pairs are separated by a colon :.
Example:
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
Adding Key-Value Pairs: You can add new key-value pairs to the dictionary.
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
my_dict["job"] = "Engineer" # Add a new key-value pair
print(my_dict) # Output: {'name': 'Alice', 'age': 25, 'city': 'New York', 'job': 'Engineer'}
Output:
{'name': 'Alice', 'age': 25, 'city': 'New York', 'job': 'Engineer'}
Removing Key-Value Pairs: You can remove key-value pairs using the del
statement.
my_dict = {"name": "Alice", "age": 25, "city": "New York", "job": "Engineer"}
del my_dict["job"] # Remove a key-value pair
print(my_dict) # Output: {'name': 'Alice', 'age': 25, 'city': 'New York'}
Output:
{'name': 'Alice', 'age': 25, 'city': 'New York'}
Accessing Elements: You can access the items of a dictionary by referring to its key
.
my_dict = {"name": "Alice", "age": 25, "city": "New York", "job": "Engineer"}
print(my_dict["name"]) # Output: Alice
# Using get() method for accessing elements
print(my_dict.get("age")) # Output: 25
Output:
Alice
25
Note: The get()
method returns None
instead of KeyError
if the key is not found in the dictionary.
4. Built-in Functions in Python
Before we dive into more complex topics, it’s essential to familiarize ourselves with Python’s built-in functions. A built-in function is a function that is always available for use in Python, without the need for any imports. These functions are an integral part of Python, designed to help perform basic tasks quickly and efficiently. Here, we introduce some useful built-in functions that you will use frequently when coding in Python:
len():
Returns the length (the number of items) of an object.
my_list = [1, 2, 3, 4, 5]
print(len(my_list)) # Outputs: 5
Output:
5
input():
Reads a line from input (keyboard), converts it to a string, and returns it.
name = input("Enter your name: ")
print("Hello, " + name) # Outputs: Hello, (whatever name you entered)
Output:
Enter your name: John
Hello, John
str()
,int()
,float()
: Convert their arguments into the corresponding type.
# define a number as an integer
num = 10
# convert the integer to a string and print
num_str = str(num)
print("String representation of num: " + num_str)
# convert the string back to an integer and print
num_int = int(num_str)
print("Integer representation of num_str: " + str(num_int))
# convert the integer to a float and print
num_float = float(num)
print("Float representation of num: " + str(num_float))
Output:
String representation of num: 10
Integer representation of num_str: 10
Float representation of num: 10.0
list()
,tuple()
: Used to convert their arguments into the respective type.
# define a string
s = "Hello"
# convert the string to a list and print
s_list = list(s)
print("List representation of s: " + str(s_list))
print(type(s_list))
# convert the list back to a tuple and print
s_tuple = tuple(s_list)
print("Tuple representation of s_list: " + str(s_tuple))
print(type(s_tuple))
Output:
List representation of s: ['H', 'e', 'l', 'l', 'o']
<class 'list'>
Tuple representation of s_list: ('H', 'e', 'l', 'l', 'o')
<class 'tuple'>
min()
,max()
: Return the smallest and largest numbers in a list or array, respectively.
numbers = [4, 2, 9, 7]
print(min(numbers)) # Outputs: 2
print(max(numbers)) # Outputs: 9
Output:
2
9
abs()
: Returns the absolute value of a number.
print(abs(-7)) # Outputs: 7
Output:
7
sum()
: Returns the sum of all items in an iterable (like a list or tuple).
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # Outputs: 15
Output:
15
round()
: Rounds a number to the nearest integer, or to the specified number of decimals if that is provided.
print(round(3.14159, 2)) # Outputs: 3.14
Output:
3.14
sorted()
: Returns a new sorted list from the items in an iterable.
numbers = [5, 1, 9, 3]
print(sorted(numbers)) # Outputs: [1, 3, 5, 9]
Output:
[1, 3, 5, 9]
These built-in functions provide some of the fundamental operations you’ll frequently use when programming with Python. They can be used directly in your code without any need for import statements.
5. Python Control Flow
Control flow in Python refers to the order in which the program’s code executes. The control flow of a Python program is regulated by conditional statements, loops, and function calls. This section will cover conditional statements (if, else, elif)
, loops (for, while)
, and control flow tools (break, continue, pass)
.
5.1 Conditional Statements:
Conditional statements help our program decide what to do based on certain conditions.
if statement:
The if
statement is like a question. It asks, “Is this condition true? If so, perform the following action.”
weather = "rainy"
if weather == "rainy":
print("Don't forget your umbrella!")
Output:
Don't forget your umbrella!
In this example, the program checks if the weather
is “rainy”. If so, it will advise you to not forget your umbrella.
elif statement:
elif
is short for “else if”. It’s used to check for additional conditions if the condition in the if statement was not met.
weather = "sunny"
if weather == "rainy":
print("Don't forget your umbrella!")
elif weather == "sunny":
print("Wear your sunglasses!")
Output:
Wear your sunglasses!
Here, the program checks if the weather
is “rainy”. If not, it checks if the weather
is “sunny”. If so, it suggests you to wear your sunglasses.
else statement:
The else
statement catches anything that isn’t caught by the preceding conditions.
weather = "snowy"
if weather == "rainy":
print("Don't forget your umbrella!")
elif weather == "sunny":
print("Wear your sunglasses!")
else:
print("Stay at home!")
Output:
Stay at home!
Here, if the weather
is neither “rainy” nor “sunny”, the program advises you to stay at home.
5.2 Loops:
Loops allow us to run a block of code several times
for loop:
A for
loop is used to iterate over a sequence (like a list, tuple, string, or range) or other iterable objects. It’s like saying, “For each item in this group, do these steps.”
The structure of a for
loop is:
colors = ['Red', 'black', 'Green'] # List itmes of colors
for color in colors:
print(color)
Output:
Red
black
Green
Here’s a brief breakdown:
for
: The keyword to initiate a for loop.color
: A placeholder for each item in the iterable. It can be anything like num, x, y, z.in
: The keyword that links the variable to the iterable.colors
: The object or collection to be iterated over. In above Its alist
name of colors:
: A colon to mark the start of the block of code to be executed.
Next Example:
name = 'Carol'
for a in name:
print(a)
Output:
C
a
r
o
l
In a for
loop, we can use the range()
function to generate and iterate over a sequence of numbers. The range()
function can take one, two, or three parameters. In its simplest form, range(stop)
takes one argument – the point where the sequence stops (exclusive). In a two-argument form, range(start, stop), it takes a starting point (inclusive) and a stopping point (exclusive). The three-argument form,
range(start, stop, step)`, takes a start point, a stop point, and a step for each iteration. Now, let’s see all these forms in action:
# Using range with a single argument.
print("Using range with one argument:")
for i in range(5):
print(i)
# Using range with start and stop arguments.
print("\nUsing range with start and stop arguments:")
for i in range(2, 6):
print(i)
# Using range with start, stop, and step arguments.
print("\nUsing range with start, stop, and step arguments:")
for i in range(2, 10, 2):
print(i)
Output:
Using range with one argument:
0
1
2
3
4
Using range with start and stop arguments:
2
3
4
5
Using range with start, stop, and step arguments:
2
4
6
8
for loop in dictionaries data types:
people_languages = {
'Alice': ['Python', 'R', 'SQL'],
'Bob': ['Java'],
'Charlie': ['Python', 'JavaScript', 'C#', 'HTML']
}
for person, languages in people_languages.items():
print(f"{person} likes:")
for language in languages:
print(f"- {language}")
Output:
Alice likes:
- Python
- R
- SQL
Bob likes:
- Java
Charlie likes:
- Python
- JavaScript
- C#
- HTML
This script does the following:
- It creates a dictionary
people_languages
where each person (like ‘Alice’, ‘Bob’, and ‘Charlie’) has a list of programming languages they like. - The
for
loop forperson, languages in people_languages.items():
iterates through the dictionary. For each iteration,person
is the name of the person and languages is the list oflanguages
they like. - Inside the
for
loop,print(f"{person} likes:")
prints the name of the person. - Another
for
loop forlanguage in languages:
iterates over the list of languages for the current person. It prints each language with-
before it.
So this code prints who likes which programming languages in an organized way.
while loop:
The while
loop in Python is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The while
loop can be thought of as a repeating if
statement. The code in the body of the while loop is executed repeatedly as long as the condition is True
.
Example 1: Basic While Loop
i = 1
while i <= 5:
print(i)
i += 1
Output:
1
2
3
4
5
Here, i
is initially 1. The while
loop checks if i
is less than or equal to 5. If true, it prints the value of i
and then increments i by 1. This process repeats until i
is no longer less than or equal to 5.
Example 2 : simple Python program that uses a while loop to continually ask the user for a number and then prints whether that number is odd or even:
while True:
number = input("Please enter a number (or 'quit' to stop): ")
if number.lower() == 'quit':
break
number = int(number)
if number % 2 == 0:
print(f"The number {number} is even.")
else:
print(f"The number {number} is odd.")
Output:
Please enter a number (or 'quit' to stop): 45
The number 45 is odd.
Please enter a number (or 'quit' to stop): 24
The number 24 is even.
Please enter a number (or 'quit' to stop): quit
5.3. Control Statements:
Control statements allow us to manage the flow of our loops.
break statement: The break
statement is used to completely exit the loop before it has run its course.
for number in range(10):
if number == 5:
break
print(number)
Output:
0
1
2
3
4
Here, the loop will stop once number
equals 5, even though the range
goes up to 9.
continue statement: The continue
statement is used to skip the rest of the current loop iteration and immediately start the next one.
for number in range(10):
if number == 5:
continue
print(number)
Output:
0
1
2
3
4
6
7
8
9
In this example, when number
equals 5, the program skips printing 5 and immediately continues with the next number.
In Python, pass
is a placeholder statement. We use it when we require for a statement to be syntactically correct but don’t want any action to be taken. It’s commonly used when we’re in the middle of writing new code and haven’t yet decided what should happen in a block where some code is expected.
Here’s a simple example of a for
loop with a pass
statement:
for num in [1, 2, 3, 4, 5]:
if num == 3:
pass # This is where we don't want to take any action
else:
print(num)
Output:
1
2
4
5
In this code, we’re looping over a list of numbers from 1 to 5. For each number, we check if it’s equal to 3. If it is, the pass
statement means “do nothing”. So, in this case, the number 3 won’t be printed. For any other number, we print it.
These concepts form the basis of Python’s control flow. Practice using these in different combinations to build a stronger understanding of how they work.
6. Functions in Python
6.1. What are functions and why are they important?
In Python, a function is a block of organized, reusable code that is used to perform a single, related action. Functions allow for code reuse, help make the code more readable, and divide complex problems into smaller and more manageable tasks.
In essence, a function is a way of grouping code together to perform a specific task and then giving that group a name. Once the function is defined, it can be used (or “called”) by its name elsewhere in the code.
6.2. Creating a Function
Creating a function in Python involves the def
keyword, the name of the function, parentheses ()
, and a colon :
. Any input parameters or arguments should be placed within these parentheses. The function code block is indented.
def greet():
print("Hello, world!")
In this case, greet
is the function name, and it takes no parameters. To call the function, simply write its name followed by parentheses:
greet()
Output:
Hello, world!
Functions can also take parameters and return a result:
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result) # Outputs: 8
Output:
8
In this example, add_numbers
is the function name, and a
and b
are parameters. The function calculates the sum of a and b and returns the result. The return
statement is used to exit a function and return a value.
7. Handling Errors and Exceptions in Python
7.1. Different Types of Errors
Errors in Python programming are common and are split into several types:
Syntax Errors: Also known as parsing errors, they are perhaps the most common kind of complaint you get while learning Python. The parser points to the line where the error was detected.
print("Hello, world) # The string isn't closed, so this will raise a syntax error
Output:
SyntaxError: unterminated string literal (detected at line 1)
Exceptions: Even if a statement or expression is syntactically correct, it may cause an error when it is executed. These are known as exceptions. Examples of exceptions include TypeError
, ValueError
, IndexError
, and so forth.
print(1 / 0) # This will raise a ZeroDivisionError, which is a type of exception
Output:
ZeroDivisionError: division by zero will not raise an error but will give the wrong result
7.2. Try, Except, Finally, and Raise Statements
Try & Except:
Python has a try
and except
statement for handling exceptions. The code that can cause an exception to occur is put in the try block and the handling of the exception is done in the except block.
try:
print(1 / 0)
except ZeroDivisionError:
print("You can't divide by zero!")
Output:
You can't divide by zero!
Finally:
The finally
statement is optional and can be used with the try
statement. The code in the finally block will be executed regardless of whether an exception occurs in the try block.
try:
print(1 / 0)
except ZeroDivisionError:
print("You can't divide by zero!")
finally:
print("This line is always executed.")
Output:
You can't divide by zero!
This line is always executed.
Raise:
You can use the raise statement to raise
an exception.
x = -1
if x < 0:
raise Exception("Sorry, no numbers below zero")
Output:
Exception: Sorry, no numbers below zero
Understanding how to handle errors and exceptions in Python can help you debug your code and make it more robust.
Remember, the above examples and descriptions are just to introduce you to the concept of handling errors and exceptions in Python. In practice, you may encounter many different types of exceptions and errors, and handling them can become quite complex.
8. Python Modules and Libraries
8.1. What is a Module?
A module in Python is a file that contains Python code. The code could be function definitions or executable statements, which you can import and use in your own programs. This helps you to organize your code in a clean way. Python provides many built-in modules.
For example, the math
module provides mathematical functions. Here’s how you use it:
import math
# Use a function from the math module
print(math.sqrt(16)) # output: 4.0
Output:
4.0
8.2. Python Libraries
A Python library is a collection of modules that you can use to perform common tasks. For example, the random
library provides functions for generating random numbers.
import random
# Use a function from the random library
print(random.randint(1, 10)) # prints a random integer between 1 and 10
Output:
8
9. Conclusion
Congratulations! You’ve successfully ventured into the realm of Python programming. We’ve explored a range of Python basics in this tutorial, but remember, this is just the beginning. Python’s versatility is key to fields like data science and web development.
This article has laid a foundation in Python, introducing basic syntax to complex ideas such as loops and exception handling. If you found this useful, you might want to explore my previous Pandas tutorial for a deeper dive into data science.
The goal here is to set beginners on a path to success in their coding journey. If you have questions, don’t hesitate to connect with me on LinkedIn. Follow me for more straightforward Python content, and feel free to share your feedback. Your learning fuels this journey!
Remember, the key to mastering programming is practice. Experiment with your own Python programs, learn from mistakes, and keep going. Happy coding!
Feel free to check out GitHub repo for this tutorial.
10. Reference
- Python.org. (2021). Python Tutorial.
- Sweigart, A. (2019). Automate the Boring Stuff with Python. No Starch Press.
- Matthes, E. (2019). Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming. No Starch Press. Available at Amazon
- Corey Schafer. (2021). Python Programming Beginner Tutorials. YouTube Playlist.
- Sentdex. (2021). Python Programming Basic. YouTube Playlist.
- Jovian. (2020). Data Analysis with Python: Zero to Pandas
- Colt Steele. The Modern Python 3 Bootcamp. Udemy